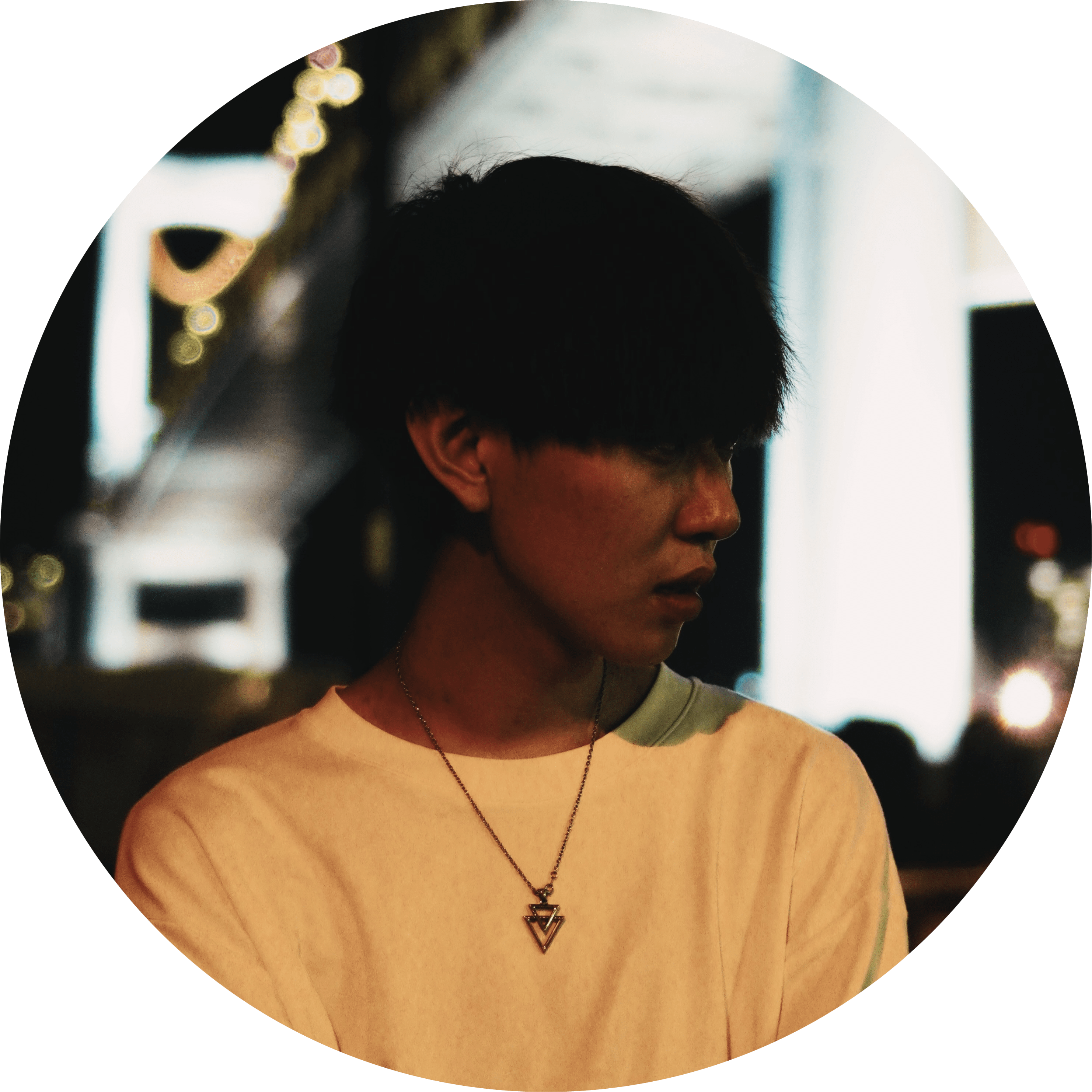
Komeno
Japanese-Brazilian software engineering student at รcole 42. Currently majoring in Japan Studies at Tokyo University of Foreign Studies. Passionate about iOS app development and language learning.
Japanese-Brazilian software engineering student at รcole 42. Currently majoring in Japan Studies at Tokyo University of Foreign Studies. Passionate about iOS app development and language learning.